צעד אחר צעד – Rabbit בשילוב עם Net.
בפוסט זה נראה דוגמא לשימוש ב-RabbitMQ בשילוב עם Net. ונממש אפליקציה פשוטה שתתחבר לתור ותשלח ותקבל הודעה,
זאת כמובן בהמשך לפוסט הקודם בו הסברתי מה זה RabbitMQ ותור הודעות
תוכן עניינים
docker run -it --rm --name rabbitmq -p 5672:5672 -p 15672:15672 rabbitmq:3.9-management
לאחר שהכל מסיים יש לכם שרת RabbitMQ מותקן שרץ על Docker, תוכלו לוודא שהכל הצליח על ידי כניסה לאפליקציה
הגדרות ברירת מחדל:
- אפליקציה http://localhost:15672/
- משתמש: guest
- סיסמא: guest
במידה והצלחתם כך נראה חלון האפליקציית הניהול של RabbitMQ:
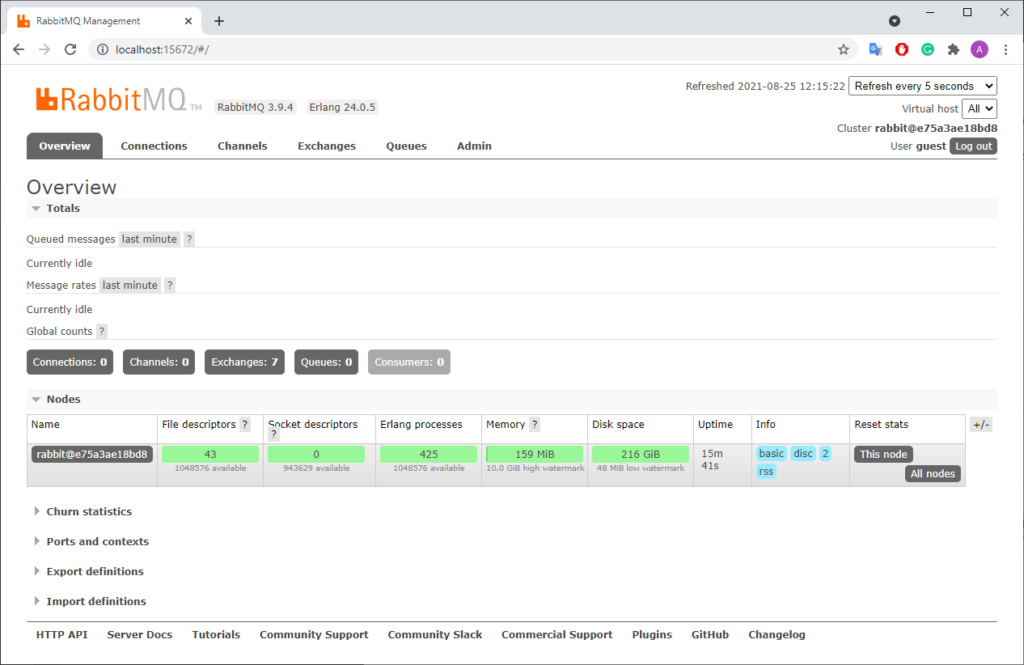
כעת ניצור שתי אפליקציות, האחת של השולח והשניה של מקבל ההודעה, ניתן לשים אותם בשני מחשבים שונים או בשני מיקרוסרביסים שונים ולתקשר עם המחשב בו יצרנו את התור.
במידה ואנו רוצים לעשות זאת ממחשב שלא מותקן עליו הRabbit נצטרך להחליף את “localhost” ב-IP של המחשב הרלוונטי.
הקוד נמצא ב GitHub או שתוכלו לראותו בהמשך הדף.
הפעלתי את הקוד בשלושה מחשבים שונים, כאשר ה1 הוא אחד השולחים לתור, כך גם מספר 2 ומספר 3 היא אפליקציה שמקבלת את ההודעות הנשלחות אל התור
השולח:
using RabbitMQ.Client;
using System;
using System.Text;
namespace Sender
{
class Program
{
public static void Main(string[] args)
{
var factory = new ConnectionFactory() { HostName = "localhost"}; // If your broker resides on a different machine, you can specify the name or the IP address.
using (var connection = factory.CreateConnection()) // Creates the RabbitMQ connection
using (var channel = connection.CreateModel()) // Creates a channel, which is where most of the API for getting things done resides.
{
//Declares the queue
var queueName = "queue1";
var durable = false; // true if we are declaring a durable queue(the queue will survive a server restart)
var exclusive = false; // true if we are declaring an exclusive queue (restricted to this connection)
var autoDelete = true; // true if we are declaring an auto delete queue (server will delete it when no longer in use)
channel.QueueDeclare(queueName, durable, exclusive, autoDelete, null);
Console.WriteLine("Please enter your message. Type 'exit' to exit.");
while (true)
{
//Converts message to byte array
var message = Console.ReadLine();
if (message?.ToUpper() == "QUIT")
{
break;
}
var data = Encoding.UTF8.GetBytes(message);
// publish to the "default exchange", with the queue name as the routing key
var exchangeName = "";
var routingKey = queueName;
channel.BasicPublish(exchangeName, routingKey, null, data);
Console.WriteLine("Sent: {0}", message);
}
}
}
}
}
המקבל:
using RabbitMQ.Client;
using RabbitMQ.Client.Events;
using System;
using System.Text;
namespace Receiver
{
class Program
{
public static void Main(string[] args)
{
var factory = new ConnectionFactory() { HostName = "localhost" }; // If your broker resides on a different machine, you can specify the name or the IP address.
using (var connection = factory.CreateConnection()) // Creates the RabbitMQ connection
using (var channel = connection.CreateModel()) // Creates a channel, which is where most of the API for getting things done resides.
{
//Declares the queue
var queueName = "queue1";
var durable = false; // true if we are declaring a durable queue(the queue will survive a server restart)
var exclusive = false; // true if we are declaring an exclusive queue (restricted to this connection)
var autoDelete = true; // true if we are declaring an auto delete queue (server will delete it when no longer in use)
channel.QueueDeclare(queueName, durable, exclusive, autoDelete, null);
var consumer = new EventingBasicConsumer(channel);
// Callback
consumer.Received += (model, deliveryEventArgs) =>
{
var body = deliveryEventArgs.Body.ToArray();
// convert the message back from byte[] to a string
var message = Encoding.UTF8.GetString(body);
Console.WriteLine("** Received message: {0} by Consumer thread **", message);
};
// Start receiving messages
channel.BasicConsume(queue: queueName, // the name of the queue
autoAck: true, // true if the server should consider messages acknowledged once delivered;
consumer: consumer); // an interface to the consumer object
Console.WriteLine("Press [enter] to exit.");
Console.ReadLine();
}
}
}
}
כל הכבוד!! אחלה מדריך! יא מלך
כתבה מעולה !
מקפיץ את הקהילה הישראלית 🙂
מה דעתך על שרת תורים במצב של קבלת הודעות מיידיות (פחות מ30 שניות )
מה החלופה בארכיטקטורה
היי גבריאל, קודם כל תודה 🙂
לא הבנתי עד הסוף את השאלה אך בכל זאת אנסה לענות.
RabbitMQ הוא בעצם שרת התורים והוא כבר מקבל את ההודעות באופן מיידי אך דואג לשים אותם בתור.
אם אנו נשלח הודעות ישירות לסרביסים בלי מתווך בדרך – מה יקרה אם אחד הסרביסים שלך רדומים? בדיוק עשה ריסט? מה יקרה להודעה?